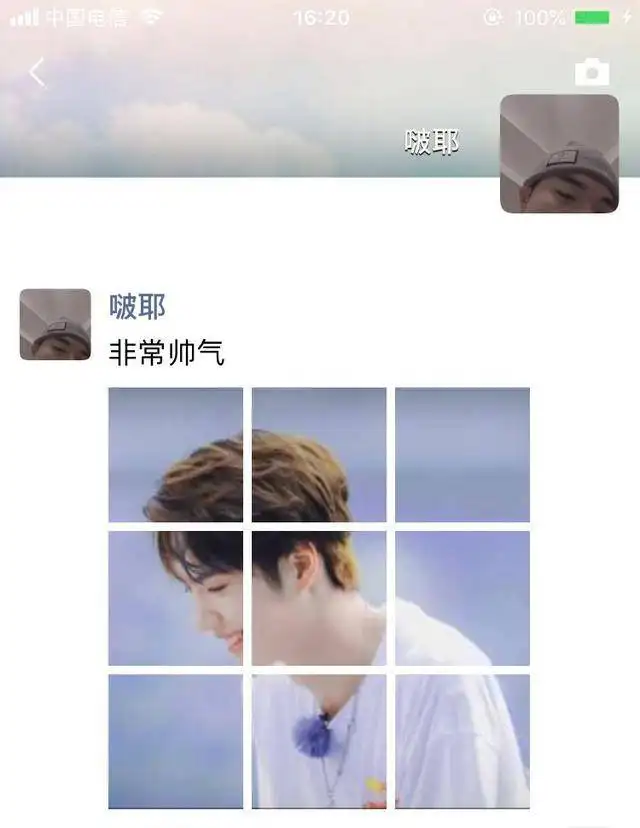
php生成九宫格图片与图片分割裁剪
生成九宫格头像(图片)
样式一:
<?php /** * 生成群组九宫格头像 * param $pic_list array 群主和前八个群成员的头像(图片地址的数组) * param $group_id int 群组id,用于生成头像名字 */ function set_img($pic_list, $group_id) { shuffle($pic_list); $pic_list = array_slice($pic_list, 0, 9); // 只操作前9个图片 $bg_w = 150; // 背景图片宽度 $bg_h = 150; // 背景图片高度 $background = imagecreatetruecolor($bg_w, $bg_h); // 背景图片 $color = imagecolorallocate($background, 237, 237, 237); // 为真彩色画布创建白色背景,再设置为透明 imagefill($background, 0, 0, $color); imageColorTransparent($background, $color); $pic_count = count($pic_list); $lineArr = array(); // 需要换行的位置 $space_x = 3; $space_y = 3; $line_x = 0; switch ($pic_count) { case 1: // 正中间 $start_x = intval($bg_w / 4); // 开始位置X $start_y = intval($bg_h / 4); // 开始位置Y $pic_w = intval($bg_w / 2); // 宽度 $pic_h = intval($bg_h / 2); // 高度 break; case 2: // 中间位置并排 $start_x = 2; $start_y = intval($bg_h / 4) + 3; $pic_w = intval($bg_w / 2) - 5; $pic_h = intval($bg_h / 2) - 5; $space_x = 5; break; case 3: $start_x = 40; // 开始位置X $start_y = 5; // 开始位置Y $pic_w = intval($bg_w / 2) - 5; // 宽度 $pic_h = intval($bg_h / 2) - 5; // 高度 $lineArr = array(2); $line_x = 4; break; case 4: $start_x = 4; // 开始位置X $start_y = 5; // 开始位置Y $pic_w = intval($bg_w / 2) - 5; // 宽度 $pic_h = intval($bg_h / 2) - 5; // 高度 $lineArr = array(3); $line_x = 4; break; case 5: $start_x = 30; // 开始位置X $start_y = 30; // 开始位置Y $pic_w = intval($bg_w / 3) - 5; // 宽度 $pic_h = intval($bg_h / 3) - 5; // 高度 $lineArr = array(3); $line_x = 5; break; case 6: $start_x = 5; // 开始位置X $start_y = 30; // 开始位置Y $pic_w = intval($bg_w / 3) - 5; // 宽度 $pic_h = intval($bg_h / 3) - 5; // 高度 $lineArr = array(4); $line_x = 5; break; case 7: $start_x = 53; // 开始位置X $start_y = 5; // 开始位置Y $pic_w = intval($bg_w / 3) - 5; // 宽度 $pic_h = intval($bg_h / 3) - 5; // 高度 $lineArr = array(2, 5); $line_x = 5; break; case 8: $start_x = 30; // 开始位置X $start_y = 5; // 开始位置Y $pic_w = intval($bg_w / 3) - 5; // 宽度 $pic_h = intval($bg_h / 3) - 5; // 高度 $lineArr = array(3, 6); $line_x = 5; break; case 9: $start_x = 5; // 开始位置X $start_y = 5; // 开始位置Y $pic_w = intval($bg_w / 3) - 5; // 宽度 $pic_h = intval($bg_h / 3) - 5; // 高度 $lineArr = array(4, 7); $line_x = 5; break; } foreach ($pic_list as $k => $pic_path) { $kk = $k + 1; if (in_array($kk, $lineArr)) { $start_x = $line_x; $start_y = $start_y + $pic_h + $space_y; } $pathInfo = pathinfo($pic_path); switch (strtolower($pathInfo['extension'])) { case 'jpg': case 'jpeg': $imagecreatefromjpeg = 'imagecreatefromjpeg'; break; case 'png': $imagecreatefromjpeg = 'imagecreatefrompng'; break; case 'gif': default: $imagecreatefromjpeg = 'imagecreatefromstring'; $pic_path = file_get_contents($pic_path); break; } $resource = $imagecreatefromjpeg($pic_path); // $start_x,$start_y copy图片在背景中的位置 // 0,0 被copy图片的位置 // $pic_w,$pic_h copy后的高度和宽度 imagecopyresized($background, $resource, $start_x, $start_y, 0, 0, $pic_w, $pic_h, imagesx($resource), imagesy($resource)); // 最后两个参数为原始图片宽度和高度,倒数两个参数为copy时的图片宽度和高度 $start_x = $start_x + $pic_w + $space_x; } //header头,直接输出到浏览器,不用就注释掉 //header("Content-type: image/jpeg"); // 第二个参数,设置图片保存位置和名称;第三个参数,设置图片质量,从0-100,默认值是75 $time = time(); $imgname = 'Uploads/GroupLogo/' . $group_id . $time . '.jpg'; $result = imagejpeg($background, $imgname, 100); if ($result != 1) { return 0; } return $time; } ?>
样式二:
<?php public function createMosaicGroupAvatar($pic_list = array(), $bg_w = 396, $bg_h = 396) { if (!$pic_list) { $result = new \stdClass(); $result->img_id = 0; $result->url = ''; return $result; } $pic_list = array_slice($pic_list, 0, 9); // 只操作前9个图片 $background = imagecreatetruecolor($bg_w, $bg_h); // 背景图片 $color = imagecolorallocate($background, 216, 216, 216); // 为真彩色画布创建白色背景,再设置为透明 imagefill($background, 0, 0, $color); //区域填充 imageColorTransparent($background, $color); // 将某个颜色定义为透明色 $pic_count = count($pic_list); $lineArr = array(); // 需要换行的位置 $space_x = 3; $space_y = 3; $line_x = $pic_h = $start_y = 0; switch ($pic_count) { case 1: // 正中间 $start_x = intval($bg_w / 4); // 开始位置X $start_y = intval($bg_h / 4); // 开始位置Y $pic_w = intval($bg_w / 2); // 宽度 $pic_h = intval($bg_h / 2); // 高度 break; case 2: // 中间位置并排 $start_x = 2; $start_y = intval($bg_h / 4) + 3; $pic_w = intval($bg_w / 2) - 5; $pic_h = intval($bg_h / 2) - 5; $space_x = 5; break; case 3: $start_x = 124; // 开始位置X $start_y = 5; // 开始位置Y $pic_w = intval($bg_w / 2) - 5; // 宽度 $pic_h = intval($bg_h / 2) - 5; // 高度 $lineArr = array(2); $line_x = 4; break; case 4: $start_x = 4; // 开始位置X $start_y = 5; // 开始位置Y $pic_w = intval($bg_w / 2) - 5; // 宽度 $pic_h = intval($bg_h / 2) - 5; // 高度 $lineArr = array(3); $line_x = 4; break; case 5: $start_x = 85.5; // 开始位置X $start_y = 85.5; // 开始位置Y $pic_w = intval($bg_w / 3) - 5; // 宽度 $pic_h = intval($bg_h / 3) - 5; // 高度 $lineArr = array(3); $line_x = 5; break; case 6: $start_x = 5; // 开始位置X $start_y = 85.5; // 开始位置Y $pic_w = intval($bg_w / 3) - 5; // 宽度 $pic_h = intval($bg_h / 3) - 5; // 高度 $lineArr = array(4); $line_x = 5; break; case 7: $start_x = 166.5; // 开始位置X $start_y = 5; // 开始位置Y $pic_w = intval($bg_w / 3 ) - 5; // 宽度 $pic_h = intval($bg_h / 3 ) - 5; // 高度 $lineArr = array(2,5); $line_x = 5; break; case 8: $start_x = 80.5; // 开始位置X $start_y = 5; // 开始位置Y $pic_w = intval($bg_w / 3) - 5; // 宽度 $pic_h = intval($bg_h / 3) - 5; // 高度 $lineArr = array(3,6); $line_x = 5; break; case 9: $start_x = 5; // 开始位置X $start_y = 5; // 开始位置Y $pic_w = intval($bg_w / 3) - 5; // 宽度 $pic_h = intval($bg_h / 3) - 5; // 高度 $lineArr = array(4,7); $line_x = 5; break; } foreach ($pic_list as $k => $pic_path) { $kk = $k + 1; if ( in_array($kk, $lineArr) ) { $start_x = $line_x; $start_y = $start_y + $pic_h + $space_y; } $image_info = getimagesize($pic_path); $image_suffix = end(explode('/', end($image_info))); switch ($image_suffix) { case 'png': $resource = imagecreatefrompng($pic_path); break; default: $resource = imagecreatefromjpeg($pic_path); } $image_width = imagesx($resource); $image_height = imagesy($resource); // 图片圆角处理 $radius = 0; //20 40-貌似不错 50也不错 // lt(左上角) $lt_corner = $this->get_lt_rounder_corner($radius); imagecopymerge($resource, $lt_corner, 0, 0, 0, 0, $radius, $radius, 100); // lb(左下角) $lb_corner = imagerotate($lt_corner, 90, 0); imagecopymerge($resource, $lb_corner, 0, $image_height - $radius, 0, 0, $radius, $radius, 100); // rb(右上角) $rb_corner = imagerotate($lt_corner, 180, 0); imagecopymerge($resource, $rb_corner, $image_width - $radius, $image_height - $radius, 0, 0, $radius, $radius, 100); // rt(右下角) $rt_corner = imagerotate($lt_corner, 270, 0); imagecopymerge($resource, $rt_corner, $image_width - $radius, 0, 0, 0, $radius, $radius, 100); // $start_x,$start_y copy图片在背景中的位置 // 0,0 被copy图片的位置 $pic_w,$pic_h copy后的高度和宽度 imagecopyresized($background, $resource, $start_x, $start_y, 0, 0, $pic_w, $pic_h, imagesx($resource), imagesy($resource)); // 最后两个参数为原始图片宽度和高度,倒数两个参数为copy时的图片宽度和高度 $start_x = $start_x + $pic_w + $space_x; } $fname = 'xshop' . date('YmdHis').rand(100, 999); $tmp_img_path = '/tmp/'.$fname.'.jpg'; imagejpeg($background, $tmp_img_path); /* 上传图片 */unlink($tmp_img_path); // 释放内存 imagedestroy($background); return $wx_img_res; } /** * [产生一个弧角图片] * @param [type] $radius [弧度] * @return [type] [description] */ public function get_lt_rounder_corner($radius) { // $radius:弧角图片的大小 $img = imagecreatetruecolor($radius, $radius); $bgcolor = imagecolorallocate($img, 223, 223, 223); // $bgcolor = imagecolorallocate($img, 216, 216, 216); $fgcolor = imagecolorallocate($img, 0, 0, 0); imagefill($img, 0, 0, $bgcolor); // $radius,$radius:以图像的右下角开始画弧 // $radius*2, $radius*2:已宽度、高度画弧 // 180, 270:指定了角度的起始和结束点 // fgcolor:指定颜色 imagefilledarc($img, $radius, $radius, $radius * 2, $radius * 2, 180, 270, $fgcolor, IMG_ARC_PIE); // 设置颜色为透明 imagecolortransparent($img, $fgcolor); return $img; } ?>
图像分割成九宫格(多张图片):
<?php function segmentation($w, $h, $filename) { //获取原图wdith和height list($width, $height) = getimagesize($filename); //分割后大小 $newwidth = floor($width / $w); $newheight = floor($height / $h); //复制 $source = imagecreatefromjpeg($filename); for ($i = 0; $i < $h; $i++) { for ($j = 0; $j < $w; $j++) { $startY = $i * $newheight; $startX = $j * $newwidth; $thumb = ImageCreateTrueColor($newwidth, $newheight); //结果对象,来源对象,结果对象x起点,结果对象y起点,来源对象x起点,来源对象y起点,需要的width,需要的height imagecopy($thumb, $source, 0, 0, $startX, $startY, $newwidth, $newheight); imagejpeg($thumb, "{$i}{$j}.jpg", 100); } } } segmentation(4, 4, "bg.jpg"); ?>